I took programming dangers in the game as an opportunity to take a breather from coding and realize some art assets.
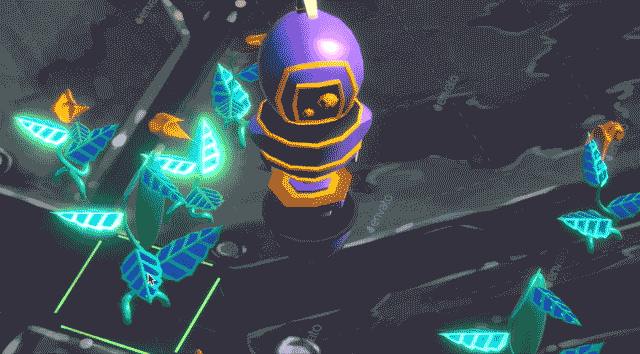
Cinematic/tech artist & filmmaker. Online since 1999.
Since I was mostly the programmer, I’d like to look at some of the systems that make the game engine work. But first…
For me, what made this game possible was an email exchange with Michael Schmidt at Unity, in which he cleared up something critical. Since Unity regards every script as a different C# type, how can you subclass a script into different variations? More specifically, how can one script interact with another when it doesn’t know if it’s the class, one of the subclasses, or one of the subclass’s subclasses?
The key is that Unity will treat a subclass script as if it were any class up its chain of inheritance. When I subclass ActualThing into Upgrade, and Upgrade into Sensor, other scripts can reference a Sensor script as if it were an Upgrade script or an ActualThing script. So these lines are equivalent:
float currentMass = someGameObject.GetComponent<ActualThing>().mass;
float currentMass = someGameObject.GetComponent<Upgrade>().mass;
float currentMass = someGameObject.GetComponent<Sensor>().mass;
A Sensor script can then override, for instance, ActualThing’s takeDamage() function, and respond to it differently than, say, a rock would:
//in ActualThing:
public virtual void takeDamage(float damageAmount){
//reduce hp
//in Sensor:
public override void takeDamage(float damageAmount){
//reduce hp
//reduce sight distance
This is how basic object inheritence patterns can be implemented in Unity.
The Grid: The first programming challenge was an infinite, non-repeating grid. Based on my previous thinking on large pseudorandom world generation, I worked out a system that places BigTiles (containing a 10×10 grid of normal game Tiles and other content on top of them) from a list of available BigTiles. A given x and y will always generate the same BigTile, allowing the game to dispose of BigTiles it no longer needs, but generate them again if needed. Each game picks a random x and y offset to the starting position–a pair of C# ints of value -2 billion to 2 billion. The x and y offsets are summed and used as another pseudorandom seed to further shuffle things around atop the BigTile. The list of available BigTiles changes based on the distance from the game’s starting point, allowing the game to gate more powerful and challenging content. Specific BigTiles can also be forced to appear, like the starting location.
Pathfinding: The bots use an A* pathfinding system. Niek worked out the initial code, and I adapted it to allow planning without executing the route (for AI reasoning) and to talk to the existing grid-based systems. This required a deep dive into Amit Patel’s A* Pages, a deep summation of pathfinding systems, which I highly recommend.
AI: The bots not being driven by the player have competing desires, to which they assign weights based on need and availability of a solution. The highest weight wins. They may reevaluate their options several times on the way to their goal, but the current “plan” has a sunk-cost-fallacy bonus attached, to reduce indecisiveness. The AI can query the Pathfinder (a separate script) for the “cost” of a path; it will also store the steps needed for pathfinding, to avoid a processing-heavy path recalculation for the action it ultimately decides to take.
Mystery Boxes: Remember how every BigTile has its own pseudorandom seed number? MysteryBoxes are a system that uses these to “randomly” shuffle things around on BigTiles when they’re generated–plants, upgrades, whatever you’d like. The plant growing on top of a toppled monument? That’s not scripted. One limitation is that if something on a BigTile gets destroyed, it’ll reappear if the player ever goes far enough away and comes back. A special subclass, the CPUBox, will generate a new CPU (the base of a new bot) if your party is smaller than the allowed size, or a random Upgrade if not. Like other gated content, the maximum number of party members increases with distance from your starting point.
“Tiny Convoy” is as a demo-length convoy simulator–a moving base builder–developed in Unity 2019 by Niek Meffert, Lucas Oliveira and myself. Playable betas for Mac and Windows can be downloaded here. The goal is to survive as a group of tiny robots in a big dangerous world: find power, upgrade your bots, and avoid dangers.
Remember the annoying escort missions in “Warcraft III,” where your soldiers kept hauling off to attack anything that moved, instead of protecting the things they were supposed to? It’s like that. Except your bots can’t attack anything. You are essentially robot herbivores. Looked at another way, “Tiny Convoy” is a herd simulator.
As a small team in our first year Masters, the work was loosly siloed. Oliveira took the lead on modelling and texturing, Meffert was point on UI and the first version of the A* pathfinder, and the C# code base is about 90% mine. (I also designed the Touch-Me-Not exploding plant.)
1. Emergent visual storytelling–
Design Pilarsno dialogue, no scripted beats
2. Keep moving & exploring
3. No weapons
4. Cooperation is essential to survive & thrive
Our goal was to marry emergence and progression in an interesting and satisfying way. We wanted to build a game in which the player could create in their own mind an emergent storyline around an algorithmically-generated world. If the content one encountered became too boring and undifferentiated, there would be no sense of progression, and little incentive to keep exploring. On the other hand, if too many things were deliberately laid in the player’s path at defined points, it would take on the feeling of an “on rails” story game, to the detriment of replayability.
Every “Civilization” player remembers when the Mongols took their capital, and they rallied back to win. What happened was only the randomized game content interacting with the rules and the player’s choices, but it took on the quality of a narrative moment because it was different and valued by the player. Likewise, we wished to create moments like “When Little Blue got stepped on,” and “When we found the field of Touch-Me-Nots on the other side of the desert”—moments created entirely by the game systems interacting with the player’s choices, but which would feel personally meaningful.
To do this, we needed to construct a series of game loops, with systems of player feedback. We would gradually challenge the player by introducing limits on stock (not enough power available) and new sinks (dangers that damage parts). The game engine would introduce new content and changes to the starting conditions in a measured manner as the player moved farther and farther across the game world.
Forest Fire is a simple Pandemic-like (or Pandemic-lite) game for 2-4 kids, age 5 and up. The players work together to put out the fires in a forest. Encourage them to talk over their moves, and strategize as a team.
Make a 6×6 grid. Number the rows and columns 1-6. These are the Forest Squares. Place a player piece for each kid around the outer edge of the grid, next to any square they choose.
Get 2 dice. These are the Fire Dice, which you will roll to add Fires (counters) to the board–one roll of the dice per player per round. Announce the rolls to the players as down then across (e.g. “1 down, 4 across.”) Have the players add a Fire counter to the square you call out. Squares can have more than one Fire counter at a time. Now explain that when there’s a non-burning (empty) square between a new Fire and an existing Fire, the Fire spreads to the square inbetween–horizontally, vertically, or diagonally. (This is like Go, only Fire can only “flip” a single empty square.) Roll once for each player, then begin.
If at the end of a round anyone is still on a square with a Fire, they’re out of the game. Disaster! If all forest squares are burning, it’s game over. When the kids put out all the Fires, they win!
Difficulty: A Firebreak is a clearing (natural or artificial) in the forest that fire can’t easily cross. With a second type of counter, you may at the beginning of the game roll to add Firebreaks to the board. Players can move onto Firebreak squares, but if a Fire is rolled on a Firebreak square, nothing happens. For an easier game, roll up to one Firebreak per player. Roll fewer or none for a challenge game. (Remember, the players still lose if every non-Firebreak square is ablaze at the same time!)
Introduce the types of player characters one game at a time:
Have the kids mime holding a firehose. Explain that a Hotshot Team is the firefighters on the ground with hoses and shovels who work to put out forest fires. (This is where the term comes from!)
A Hotshot gets 2 moves per round. Each move can be either: 1) Putting out the Fires (removing all Fire counters) from their own or an adjacent square (N, S, E, W or diagonally), or 2) Moving 1 square N, S, E or W. Moving one square off the board, like at the beginning, is allowed.
To begin the game, explain that it takes time to get Hotshot Teams to a forest fire. Because of this, the fire has time to spread. Add 2 rounds’ worth of fires to the board (one roll for each player x2) then have the players begin.
Have the kids mime holding onto the straps of a parachute. Explain that a Fire Jumper parachutes into the forest near a fire. Because of this, they can’t carry as much equipment, but once they hike out they can jump in again anywhere they’re needed.
A Smoke Jumper gets 2 moves per round. Similar to the Hotshot, each move can be either: 1) Putting out 1 fire (removing only 1 counter) from their own or an adjacent square (N, S, E, W or diagonally), or 2) Moving 1 square N, S, E or W. They also have a special ability: Upon moving off the board, they can “jump” to any square on the board. (Moving off the board and jumping count as a single action.)
Add 2 rounds’ worth of Fires to the board, then have the players begin.
Have the kids make an airplane with they hand (palm flat, index and ring finger together underneath the middle finger, pinky and thumb straight out to the sides). (If this is too hard, just pretend to be holding the flying yoke.) Explain that Pilots fly seaplanes which land on a body of water, fill a large tank, and then dump the water directly onto a fire.
Movement-wise, the pilot works a little differently. Every other turn, they must leave the board to refill their water tanks. Thus, every second turn, the players pick up their piece and hold it in their hand. The next turn, however, they can put out all the fire on any 3 squares in a row (N, S, E, W or diagonally).
Add 2 rounds’ worth of fires to the board. When the kids begin, remind them that they must first leave the board to fill up–so there will actually be 3 rounds of Fires added before they can begin putting them out!
Now we put the pieces together. Let the kids decide (and encourage them to discuss strategically) what each would like to be: a Hotshot, a Smoke Jumper, or a Pilot. Explain how fighting forest fires is a team effort, with people in different specialties doing different jobs. (Now we understand the pantomiming; it’s to keep everyone’s role straight!)
Add 2 rounds’ worth of fires to the board, and have the kids begin!
Thanks to some great playtesting with my nephews, please find an improved version 2 of the game here.
Forest Fire is a simple Pandemic-like (or Pandemic-lite) game for 4-6 kids, age 6 and up. The players work together to put out the fires in a forest. Encourage them to talk over their moves, and strategize as a team.
Make a 6×6 grid. Number the columns 1-6 and the rows A-F. These are the Forest Squares. Place a player piece for each kid around the outer edge of the grid, next to any square they choose.
To add Fires (counters) to the board, you will roll two dice. Announce the roll to the players as: The number from the first die, and the letter corresponding to the second die: A=1, B=2, C=3, etc. Have the players add a Fire counter to the square you call out. Now explain that when there’s a non-burning (empty) square between a new Fire and an existing Fire, the Fire spreads to the square inbetween–horizontally, vertically, or diagonally. (This is like Go, only Fire can only “flip” a single square.) Repeat once for each player, then have the players begin the round.
If at the end of a round anyone is still on a square with a Fire, they’re out of the game. Disaster! If all forest squares are burning, it’s game over. When the kids put out all the Fires, they win!
Difficulty: A Firebreak is a clearing (natural or artificial) in the forest that fire can’t easily cross. With a second type of counter, you may at the beginning of the game roll to add Firebreaks to the board. Players can move onto Firebreak squares, but if a Fire is rolled on a Firebreak square, nothing happens. For an easier game, roll one Firebreak per player. Roll fewer or none for a challenge game. (Remember, the players still lose if every non-Firebreak square is ablaze at the same time!)
Introduce the types of player characters one game at a time:
Have the kids mime holding a firehose. Explain that a Hotshot Team are the firefighters on the ground with hoses and shovels who work to put out forest fires. (This is where the term comes from!)
A Hotshot gets 2 moves per round. Each move can be either: 1) Putting out a Fire (removing a counter) from their own or an adjacent square (N, S, E, W or diagonally), or 2) Moving one square N, S, E or W. Moving one square off the board, like at the beginning, is allowed. No square can have more than one player on it at a time.
To begin the game, explain that it takes time to get Hotshot Teams to a forest fire. Because of this, the fire has time to spread. Add 2 rounds’ worth of fires to the board (one roll for each player) then have the players begin.
Have the kids mime holding onto the straps of their parachute. Explain that a Fire Jumper parachutes into the forest near a fire. Because of this, they can’t carry as much, but once they hike out they can jump in again anywhere they’re needed.
A Smoke Jumper gets 2 moves per round. Like the Hotshot, each move can be either: 1) Putting out a fire (removing a counter) from their own or an adjacent square (N, S, E, W or diagonally), or 2) Moving one square N, S, E or W. Because they have less gear, however, Fire Jumpers can only put out 1 fire per square. They also have a special ability: Upon moving off the board, they can “jump” to any square on the board. (Moving off the board and jumping count as one action.)
Add 2 rounds’ worth of Fires to the board, then have the players begin.
Have the kids make an airplane with they hand (palm flat, index and ring finger together under the middle finger, pinky and thumb out to the sides). (If this is too hard, just pretend to be holding the flying yoke.) Explain that Pilots fly modified seaplanes which land on a body of water, fill a large tank, and then dump the water directly onto a fire.
Movement-wise, the pilot works a little differently. Every other turn, they must leave the board to refill their water tanks. (The players each pick their piece up and hold it in their hand.) The next turn, however, they can put out all the fire on any 3 squares in a row (N, S, E, W or diagonally).
Add 2 rounds’ worth of fires to the board. When the kids begin, remind them that they must first leave the board to fill up–so there’s actually three rounds of Fires added before they can begin putting them out!
Now we put the pieces together. Let the kids decide (and encourage them to discuss strategically) what each would like to be: a Hotshot, a Smoke Jumper, or a Pilot. Explain how fighting forest fires is a team effort, with people in different specialties doing different jobs. (Now we understand the pantomiming; it’s to keep everyone’s role straight!)
Add 2 rounds’ worth of fires to the board, and have the kids begin!
Some call it originality. I call it being the first loser.
The iOS gaming space is crowded. Metacritic lists 10,934. Doing a word frequency analysis on their titles, we can come up with the ultimate must-hit games. I’m expecting a 20% cut when these become reality, on top of whatever Apple’s charging.
War Game Man — It is only a game, isn’t it? Man?
These are the first three nouns on our list. In order. War (349), Game (332), and Man (308), have almost a thousand hits between them, when you exclude two-letter combinations and common hyphen-ation frag-ments. More importantly, they’re three things hardcore gamers crave: Wars, games and men. So I’m told. Felch out literally anything with a tank, a guy in a helmet, and this title, and sit back until you hear the backup beep of the money truck.
Ace Ball Age: Bat Hero — The fate of Baseballalandia teeters on the edge, in this exciting sports/RPG hybrid!
Next up–still in order, without skipping any words–is a guaranteed money spigot. Ace (246) Ball (212) Age (210) Bat (207) Hero (198). “Oh gee, we don’t have any assets for a baseball title or an RPG,” SAID NO ONE EVER! You’ll launch the project Tuesday and be on the app store by Friday. Buy some Sponsored Content (“This Baseball RPG Will Make You Blot Out the Sun With Jizz–Especially If You’re a Woman!”) and start looking like a hoarder’s house but with money stacks.
Tar/Ash Ant Art — Navigate your half-poisoned ants through a ruined canvas factory–then sell your canvases on the fickle, tasteless modern art speculation market!
Sure, Tar (198) Ash (197) Ant (193) Art (188) is probably 90% pieces of other words, but it’s also in order, and also (while a little dark) the sort of strange-sounding, boring-to-play game that smartphone gamers love to hype. Start the train early, with forum posts, concept art, and “first looks.” Maybe put out an unplayable alpha to presale backers (basically the game with a broken start button), an almost-playable beta (a working start button, no levels, and a “crash app after 80 seconds” timer), and finally–finally–the big release (the alpha, with a better splash screen). Go Free to Play after three months. Charge $10 to stop the app from filling your Notification Center with achievement trophies and dead ants.
Maybe not a sure hit now, but they’ll clamor for a VR remake in 12 years.
You want longer words? How about this:
King Venture Adventure — The game that we reskinned with baseball assets to make Ace Ball Age: Bat Hero.
Two for one! King (187) doesn’t even come up in that many other words, and while Venture (184) technically is included in every instance of Adventure (179), they’re still the next three nouns on our list–and still in order, with no gaps. Adventure ho, Your Majesty! Ho I say!
Don’t want to use any more three-letter words at all? Fine. We can still play:
Star Zombie — Star. Zombie. It was on the tip of everyone’s tongue. We just gave it a name.
You got chocolate in my edible body paint, peanut! Star (177) Zombie (170) finally brings together two things the market isn’t at all sick of: Zombies, zombies, zombies, and games with vague generic sci-fi backdrops. Oh no please. Keep some of your money. I have so much…
Battle World: Super Land — Only one can dominate something, somewhere. Presumably!
They said it couldn’t be done in an iPhone game, but we made a game about war! And we made it with the next few words, in order, with no gaps. In Battle (165) World (152) Super (149) Land (147) you decide how to wage peace across the ill-fitting board, in the only game to include square, hexagonal, pentagonal, and rhomboid tiles in the same otherwise largely undifferentiated levels.
Aw hell, just give me $1MM now and I’ll throw in the next 21 (in-order, four or more letter, without gaps, guaranteed hit titles) for 10% off the top:
Word Puzzle Monster — (146, 139, 133) More puzzles! More monsters! Less attention span! HEYYY-AAAH!
Space Quest: Drag Night — (128, 123, 116, 113) Not all space heroes wear capes, but when they do, they’re velour.
Dragon Cape — (108, 104) Not all dragons wear velour, but…
Edition Edit — (97, 97) It’s 1933. You’re William Allan Neilson and Thomas A. Knott. Can you finish the Webster’s New International Dictionary?
Dead Escape Time: Episode Legend — (95, 94, 90, 88, 88) Not only is this probably edgy in some way, but say “episode” and your DLC levels become full-priced sequels.
Last Rush Defense — (87, 87, 80) Tower defense has never been this towery, or defensive!
Fight Racing: Tiny Story (Mini) — (80, 78, 77, 74, 74) Kart man, do!
Dark Jump — (74, 74) You’ll fall. A lot. The paincore hit of the month of Maying.
Light Race Soccer — (68, 68, 68) All Soccer Players Matter.
Pocket Craft Fish — (67, 63, 62) Maybe crafting has nothing to do with fishing, but maybe shut the hell up.
Rock Rain Tale — (61, 61, 61) “Sit here by the fire, promising indigenous youth, and I’ll tell you the tale of the day the rocks rained down…”
Dash Knight Ventures — (61, 60, 58) Sadly, nostalgia-buying an even worse knockoff of Rocket Knight Adventures probably won’t be the worst life decision your customers will have made.
Little Plan: Lost Robot Adventures — (58, 58, 58, 58, 57) So cute it won’t matter that the game is 90% walking across different backdrops.
Kingdom Port — (56, 56) Build your… port! In the kingdom! Kingdom Port!
Fantasy Magic: Kill Less — (55, 55, 55, 54) Really, you’ve been going a bit mad with power lately. We’re all concerned about you.
Part — (54) Spread things and stick other things into places in this puzzling–and completely non-erotic–puzzle game.
Ever Fall — (54, 54) Messianic sci-fi, surreal action puzzler, or primetime WB drama? Yes!
Block Tower Island — (53, 53, 51) The sequel to Last Rush Defense! Virtually indistinguishable.
Bird Ride: Blast Legends — (51, 51, 51, 50) I’ll give you something to be angry about.
Road Shoot — (50, 50) Finally! Mayhem involving roads.
Sword Pixel Runner: Monsters Down! — (50, 50, 50, 49, 49) Save the things from the other things, in the ultimate pulse-pounding ironic hipster 8-bit whatever.
Make cheques payable to the management.
If you have a chess board, you can play chess or checkers. If you have a deck of cards, you can play any number of games. But if you have a Carcassonne set, you can only play Carcassonne. As every modern geek has one, I thought we needed another game to play with it.
You play the aunts and uncles of the polymath Verona in the dangerous and romantic city states of classical Italy. Verona is brilliant, scatter-brained, obsessive, creative, charming, a magnet for trouble–and all of 17 years old. Having promised her dearly-departed parents to look after her, it’s up to you to do the legwork of the latest mystery she’s stumbled upon.
Four players cooperate to build a board of Carcassonne tiles while trying to locate and return Clue Tokens to the starting tile. Card value based Encounters hinder you and advance the game.
Shuffle the deck of cards, including the two jokers. Find the “all city” Carcassonne tile–the Old Town, where Verona lives–and place it in the center of your play surface. Deal each player an Identity card face up. Each player selects a meeple color, placing one of that color on the starting tile, and one on their Identity card. Deal one additional card–the Clue Card–face-up beside the deck, and put a meeple of the unused color atop it as a Clue Token. Randomize the remainder of the normal Carcassonne tiles and set them aside. Randomize the River tiles, and prepare to start with them.
Each card in play represents a unique person. Your Identity card is your player character. The face value represents a person’s role in the social hierarchy, from the cutpurse and con artist (2-3) through the city leaders and geniuses (J, Q, K, A).
Card suits represent specialties:
Play proceeds clockwise from the dealer. Each turn has three phases:
At the beginning of a turn, the player always attempts to place a tile–either a face down tile, or an unplayed (face-up) tile. Tile placement rules are identical to normal Carcassonne (including the need to start with the River tiles) except for one major change: A tile must be placed on a space adjacent to the one your meeple currently occupies, horizontally, vertically, or along a diagonal.
If you place a tile with a shield (pennant), or complete a city, you have made a Friend. Draw a card from the deck and place it beneath your Identity card, sight-unseen. (Note that you don’t make a Friend from drawing a shield tile, only from playing it!)
If a tile can be played, it must be. You may not select a face-up tile that can not be played, but nothing requires you to select a playable tile if face-down tiles remain.
If a tile can not be played, it is placed face-up beside your Identity card, and an Encounter is triggered for the next player: Draw a card from the deck and hand it to the next player.
Danger and intrigue are ever present! Clearing Encounters is the second phase of a turn. When dealt an Encounter card, place it above your Identity card. You may not move until all your Encounters have been cleared. If you are on the same tile as a player with an Encounter card, you may help clear one of theirs. You clear an Encounter by bringing to bear a higher total value than the Encounter card itself.
You determine your total strength (value) in an Encounter as follows:
The wealthy and famous are at their strongest in the Old Town, while the lower classes are better out in the hinterlands. To find your Distance Bonus, count your current distance from Verona, either horizontally or vertically (whichever is greater).
Your Identity card’s suit is your specialty. In an Encounter against the same suit, your face value (plus or minus any Distance Bonus) is doubled. For example:
In an Encounter, you may optionally call on a Friend if you have one available. Turn one of your Friend cards over, and add its value to your Encounter. (Your friend gets no Bonuses or Multipliers). Regardless of whether it helps you clear the Encounter or not, the Friend card is then discarded.
Some Encounters include a Clue Token, which goes to you after the Encounter is cleared, and must then be returned to Verona.
At the start of the game, the first Clue Card was turned over, and a Clue Token (meeple of the unused color) was placed atop it. The Clue Card is what Verona knows about the person who holds the next piece of the puzzle. She’s never quite right.
When an Encounter is triggered with a Clue Card in play, the suit and value of the Encounter card and Clue Card are compared. If either the suit or face match, the Clue Token becomes the prize of the Encounter. Discard the current Clue Card and move the Clue Token onto the Encounter card. When you clear this Encounter, move the Clue Token onto your Identity Card. Your objective is now to return it to Verona.
Clue Tokens may be handed off from one player to another on the same tile at any time. When the Clue Token is returned to Verona’s tile, stack it atop the others, draw a new Clue Card, and place a Clue Token on it. Having received the clue and figured out what it means, Verona dispatches you to find the next piece of the puzzle. You (and Verona) win the game by collecting all of the Clue Tokens in play.
On clearing an Encounter, discard the Encounter card. If you have no more Encounter cards, you may proceed to the Movement phase. If you still have Encounters uncleared, your turn is over.
In the third and final phase of a turn, the player may move their meeple from tile to tile (horizontally or vertically) by one of the three methods below. The player may elect to start from any terrain feature (road, city or field) on their starting tile.
In city or road movement, terrain barriers may not be crossed. Thus, you may not “jump” from one city to another across a stretch of farmland, even if both city walls lie on the same tile. Rivers, likewise, may only be crossed at a bridge or at a River’s beginning or end tile. (Be sure to place your meeple on the correct bank.)
If at any time in play a Joker is drawn, all Clue hunting halts. Cover any Clue Card in play with the Joker. Young Verona has fallen head over heels for some flashy young fool, losing track of everything else, and one of the players must return to talk her out of it. The Clue Card is out of play until a player returns to the starting tile, at which point the Joker is discarded, and normal play resumes.
The conspirators are trying to box you in. Thus you lose if:
Verona must put together all the pieces of the mystery–with a little help from her family. Thus you win when:
Dumped, like the browser-based kart game, so that I may free up some synapses.
The Idea:
The Unusual Bit:
The Story:
The Art:
The Gameplay:
Character Mechanics:
Falling Down:
Levels:
The Women:
The Alchemist
Why It’s a Bad Idea:
You arrive in the town. You check into an inn. It’s on a back street. Out of the way. You’re wanted criminals, so best not to draw attention to yourselves.
You’ve been trying to find Tintmere since the airship crash. She should be in this town. But where?
Porcelain and little Night set off with a shopping list. You and Kell head upstairs.
There’s a window. The room overlooks an equally rickety row of buildings. Fourth floor. Lots of crisscrossing clotheslines and rising steam, people milling about below. In the distance over the rooflines: the Lightning Tower. Your ultimate goal.
Concrete pebbles fall discretely into the drowned, weedy flower pot in front of you. You crane, look up.
A bounty hunter tromps silently across the rusty pipes on the roof. The shadows of two more flit between the eaves.
You lean back in, smile, head gesture to Kell. A row of shurikens materialize in his hand, and he melts into the shadows. Hazard another glance out.
There’s a bamboo-like pole caught between your building and the one across the street. One floor down. It looks tenuous. But you have been working on your balance.
The next room? The walls can’t be too thick.
Nah. More fun to hide in the ratan basket.
Moments later, light feet land on the windowsill. Simultaneously, the door flies off its hinges. Two bounty hunters race into the empty room. They look around, walk to the center of the room. Suddenly a basket and a shadow burst to life, and both bounty hunters are flung out the open window with hardly a cry.
The ceiling caves in. It’s time for the big daddy bounty hunter. You exchange blows, and are both parried and thrown back. Not good. He hasn’t even broken a sweat. You grab Kell, flip him up onto the roof and climb out. The wall explodes. You make a grab for a drain pipe, swing out across sickening open space, and — Kell’s throw line jerks the pipe up toward the roof. You land. Smile. And RUN!
Rooftop chase, as the overpowered bounty hunter hurls force blasts after you, shredding the ancient stone. Chickens squawk. Cisterns topple. An adorable little girl tends a lovely three foot square rooftop garden; you scoop her up as you run by, and apologize, as the bounty hunter smashes her four flowers.
You give the girl to Kell, saying you’d like to try something. You insult the giant. A lot. Kell breaks left, sliding down the side of a building. You break right. It worked! He’s following you.
Crap.
Fight! Fighting doesn’t work. Escape! He catches you in midair. The bounty hunter sneers that the fee still gets paid if all your limbs have been pulled off. This is it.
Shwunk! The bounty hunter shakes you, looks around indeterminately. He reaches back. A magic dagger wrapped in lace protrudes from between his shoulder blades. He topples, turning to wood. The wood bleaches, hollows, cracks, shatters — poof! Nothing but dust.
You pick yourself up, squint into the sun. A lace-adorned figure steps toward you through the haze, waving. Tintmere!
—–
Now imagine that the preceeding had been generated: The overall plot. The long separation from a comrade. The clues that led you to her. The streets. The repetition of the larger goal. The foreshadowed tip-off at the flower box. The personally appropriate strategy options. The easy mini-bosses. The unstoppable mega boss. The setback getting onto the roof. The dramatic save. The comic timing. The race. The moral choice. The losing battle. The last minute save, leading into the storyline completing reunion.
As flashy as today’s RPGs are, they’re still not true Role Playing Games. In them, players are rewarded for figuring out how the game engine works and finding ways to best it, not — as the name would suggest — for immersing themselves in the role of the character.
How does a game engine implement literary devices? How do you reinforce the players’ choice to have more fun with the story, rather than the choice to simply learn better chess positions? Printed paper+pencil+friends role playing games have invented some interesting story game concepts, but digital RPGs still rely largely on grinding in the final analysis.
I’m not suggesting that hard work shouldn’t bring character improvement, but I sanction it only because that too is a literary device. It’s not, however, the only literary device. In the early days of computer games, perhaps it was the only trope that could be realistically implemented. Are we at the end of the beginning of computer games yet?
Those looking for something a little more crunchy may enjoy my RPG Stats Comparison Chart.
A comparison of the stats used to define a character across eleven popular videogame and pencil-and-paper roleplaying games. (20k PDF)
Not included on the chart are depletable scores. Each game seems to have a concept of Hit Points, a number representing the character’s moment-to-moment health, with the possible exception of outlier EVE Online and it’s complete lack of physical traits. Most games that invoke magic of one sort or another have a rechargeable score representing the total amount of magic which may be invested in an action at any given time. Wealth is typically also a depletable score.
All games surveyed also deal with situational bonuses. These may be weapons and armor, single-use or depletable items, or learned skills. Even games with simple stat structures like Shining Force II create highly varied play structures using such bonuses.
Being essentially combat-based, none of the games surveyed had more than one social stat, and the majority had none. For those that did, it was always “charisma” — an ability to gain tangible favors from others. Combat-free games like Harvest Moon may deal more fully with a character’s social aspects, but as a component of adventure storytelling it appears tellingly neglected.
Literally three steps in, I’m defeated by a low gate. I’m looking down at it. I could step over it. Still I’m trapped.
I have chosen to begin this essay with a digression.
You know those programs that change the screen resolution when they go to full-screen, and then tell the other applications that they’ve done it? It’s a kind of a slapdash Mac port thing. You quit, and every window on the screen has been squished down to an absurdly wee size and moved to the top left corner of the screen. Myst V is like that.
Ultimately, that’s not the point of this essay, though.
As with Myst [I], the exploded box of which hangs on my wall for inspiration and which I can still play under Classic, the default navigation system of Myst V becomes bothersome after a few minutes. You’re rarely quite looking where you want to be, and yet with the Obsidian/Burn:Cycle-style smoothly eased tracking shots between nodes replacing Myst’s hard cuts and simple transitions, you often find yourself looking at the interesting object during movement, only to have your camera jerked away from it as the move completes. Nodes that would appear to give access to nearby areas are often a few feet from the ones that actually do. Myst V, of course, adds two additional movement styles, a free movement mode that doesn’t appear to support game pads, and a “Classic Plus” which slaves the view rotation to the mouse cursor — something not to be attempted without a truly boss frame rate and/or a love of vertigo.
Still, we haven’t reached our ultimate point.
The engine underlying Myst V is unique to the in-storyline Myst franchise. Instead of prerendered stills (Myst, Riven) or prerendered VR panoramas (Myst III, Myst IV), the game is rendered in realtime 3D. The appeal of this method for the developers is obvious. Rather than setting up shots, rendering, tweaking, rerendering, overlaying animations, and then having to move to a wholly separate software system to construct the game play, development goes straight from modeling to game engine in one step, assisted by a glut of available off the shelf software. Besides that, it just feels more modern — an unremarked upon motivation among middle-aged tech developers like the Miller brothers.
The trouble is, it’s not better. Compare the following screen shots:
Even with the best video card, which you don’t have, and all the light baking and optimization tricks in the book, the graphical quality of a frame rendered in one 30th of a second is never going to achieve the richness of a frame rendered over half an hour. The underlying models and textures must be smaller. The lighting system must be simpler. Even with a compressed color palette and knife-sharp shadows (not entirely undesirable for direct sunlight), not to mention eight years between them, the Riven screenshot is more realistic than that of Myst V. The objects are more complex and more numerous, the textures are high enough quality to be invisible, and everything that should cast a shadow does.
Of course, the quality of a still image isn’t the end of realism, which is where we uncover one of the most compelling reasons for the move to realtime 3D: Myst V moves. Look at the water in the game’s reversed wood between the worlds starting point, and even on low texture quality you’ll see ripples. Moving ripples. No more strange, frozen glass oceans. Stand still when you arrive on the beach. The clouds move slowly through the sky. The waves roll in and fall away. Birds flit through the sky (though most seem to be part of the static landscape). The sense of immersion is heightened, until, of course, you start wishing your video card could smooth those jaggies without the frame rate tanking, you notice that the smoothness of objects’ faces becomes angular at their edges, and you’re just plain stopped while floating along like Professor Xavier by a tumble of small, ordinarily fun to climb rocks.
It’s still not our point, but it’s worth mentioning that it’s best to make an insurmountable obstacle insurmountable. Personally, I can also climb a ladder with one hand, wade, and swim — not that it matters.
We do in fact have a point, and we’re getting dangerously close to it.
Myst V, while perhaps as good a puzzle game as its predecessors, has abandoned its roots. This, in itself, would not be a bad thing if the result were actually worth the change.
Let’s review what Myst V has gained:
Now let’s review what Myst V has lost:
Myst V is the last Myst game, but it need not be the death knell of the genre. There is room to move forward with the graphical adventure, while learning from the mistakes of Myst V. Realtime 3D graphics simply aren’t good enough. Is there a better way? I would suggest that there is.
First, though, review this QuickTime VR panorama of a node in Myst V. The panning doesn’t quite feel right. I suspect that there are two reasons for this, one trivial and the other quite complex.
Regarding the simpler problem, real life lenses are rarely perfectly round. They tend to flatten in the middle, creating lower distortion near the center of the image and higher distortion toward the edges. We’ve come to expect this. VR panoramas, which typically distort and display a portion of a single 360 degree image, are, I suspect, correcting for an idealized lens. Distortion is lowest at the center and increases toward the edges at a rate that is mathematically “right,” but less complex than that of a typical lens. If I’m right, this should be easy enough to overcome by tweaking the lens correction algorithms.
The second problem is more complex, and it has to do with the way cameras are actually manipulated. Photographing panoramas requires the purchase or construction of a custom camera mount which places the lens directly in the center of rotation. This is unusual. Usually, the camera is mounted at its base, placing the lens above and in front of its center of rotation. Panning thus introduces a small movement to the camera’s view position in addition to its orientation. Your eyes are likewise mounted above and in front of their center of rotation. The effect is most noticeable when objects are close-up. Close one eye and hold a pen up in front of you. Turn your head left and right. You can see different things behind the pen based on where your head is turned. You expect to. It’s part of your sense of depth, and it’s something that VR panoramas completely fail to reproduce.
Here we have arrived at our point.
I propose that a slightly novel game engine can overcome the limitations of both VR panoramas and realtime 3D in the graphical adventure genre. We can regain the detail of prerendered scenery and filmed actors without sacrificing the ability to animate portions or all of a given scene. If we accept the primacy of the node to the genre, discarding arguably unnecessary tracking shot transitions and “free” movement modes, we can consider a new style of node construction I unceremoniously dub the Dented Ball.
The Dented Ball is a real ball, or rather a very close approximation made up of several hundred triangles. Inside it lies a virtual camera, slightly above and forward of center. On the inside of the ball is mapped a high resolution image of a prerendered scene. Looking outward, the virtual camera records a portion of the scene, corrects for lens effects, and sends the resultant view to the player. This ball is our basic game node.
The scenery images, not to mention the tiny amount of data needed to construct this particular ball’s geometry, are loaded from the game DVD while the player is at a nearby node. Priority is given to the nodes directly connected to the previously occupied node, with priority further given to those portions of the landscape that the player would see first upon stepping into a given node from the previous. Nodes far behind are discarded, and reloaded only when the player nears them again. The goal is to minimize or eliminate waiting time between nodes.
Animation such as waves, birds, and even people may be added to the scene by mapping movie clips, rather than still images, to a portion or all of the inside of the Dented Ball. Modern video compression algorithms nearly half the amount of data that must be pulled off the game DVD to equal the image quality of a standard movie DVD, and modern computer DVD drives are capable of reading data much faster than is necessary to play movies compressed the old fashioned way. By feeding movie clips into the priority system above, waiting time between nodes can still be kept to a minimum. In addition, a standardized set of tools for fading, overlaying and cutting between still images should be integrated to allow for such simple effects as lightning flashes and the slow dimming of the sun as it goes behind a cloud, without requiring a large and unwieldy video clip.
In an ideal scene, there are no objects near the camera. (This is of course unlikely.) The edges of the ball on which the scene is painted are too far away from the viewer for the slight position offset of the camera to be noticeable. This would be a scene of the player floating high in the air — on the whole not very useful.
Nearby objects are the reason we call this the Dented Ball. Imagine that the player is standing near the corner of a wall. Panning right, more of the right side of the wall becomes visible, panning left, the opposite. In order to simulate this effect, the ball itself has been dented inward, toward the camera, so that its edge matches up with the wall’s corner in the prerendered scene. From the outside, the ball would appear to have a large dent in it, hence our name for it. Because the camera offset is most noticeable when objects are close, gradually falling off to imperceptibility as objects move farther away, the actual dent of a perfectly right-angled wall in the ball would not have straight edges, but would in fact taper out at an increasingly gentle angle before plunging smoothly back into the outside surface of the ball.
Despite our name though, dents aren’t the only method we’ll need to produce proper foreground/background separation while panning. (We should just go ahead and call this separation “parallax effects.”) Reference the pen with one eye closed again. It, like a blade of grass, glasses on a nearby table, and any number of other real world objects exhibit a complete separation from their background. In such cases we’ll need to slice the ball into concentric layers, like an onion, and map a series of cutout portions of the scene onto each. There will be times when we’ll need to combine slices with dents. Depth maps, grayscale images representing simply how far any point in a given image is from the camera, can be rendered from any 3D animation package, and can be used to assist an automated workflow for making these dent and layering decisions.
The Dented Ball allows us to create a richer visual experience, both static and in motion, than any previously conceived graphic adventure engine. By repurposing modern video cards to draw concentric near-3D nodes, we find a new way to leverage the technologies users and game developers already possess. We unify the rich legacy of graphic adventure games like Myst V while discarding our detrimental modern preoccupations. In doing so, we glimpse a third path though the complexities of contemporary game design and begin once again simply to explore.